Check out my GitHub repository if you want to dig into the source code directly.
WCF (Windows Communication Foundation) has served us for a long time when it comes to talking to many LOB systems (SAP etc.). You might have love or hate (though if I have to guess, it would be on hate side) relationship with WCF but it works and at times we don't have any other choice. If you happen to be in a situation where you are talking to legacy systems via WCF, you most likely have a built an API layer on top of it to serve your clients.
I was recently looking to consume WCF services using .NET Core (with the intention that I can migrate my web application to .NET Core). Very quickly I came up to this GitHub repository which was awesome. However, this .NET Core implementation is not in parity with the full framework yet. I tested it and it worked just fine with standard bindings (
As far as my needs (this particular scenario) are concerned, I couldn't get it to work for my setup. This is how the setup looks for me.
The web application (hosted in Azure Web App) talks to WCF Service (running on-premises, hosted in IIS) via Azure Service Bus Relay (in Azure)
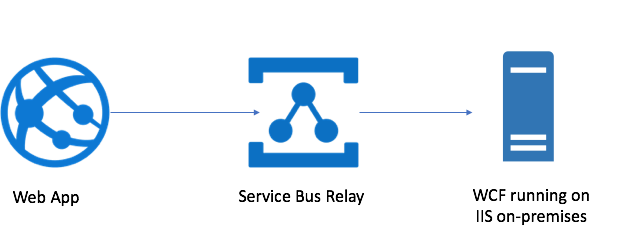
So basically, there is no equivalent to basicHttpRelayBinding
After discussing with a friend of mine, I got a suggestion to try it out with SOAP instead and it seems to work fine! I know it sounds a bit ironic that on the one hand I'm moving to the latest (and greatest) framework for my web application but at the same time, I'm going one step back and using SOAP protocol to make it work - well such is life. I would very much appreciate if anyone can point me to the better solution. but until then, let's continue forward.
So, in this blog post
So this is what
- Create Azure Service Bus Relay (read here how to create this)
- Create a WCF Service (with Azure Service Bus Relay Binding) and publish this locally to run it on IIS
- ASP.NET Core Web Application calling to WCF Service using HTTP Post SOAP request.
Follow the link I have provided above to create the service bus relay. Let's start with WCF Service. Here is the sample WCF
[ServiceContract]
public interface ICustomerService
{
[OperationContract]
string GetCustomerData(string customerId);
}
When you browse this service from IIS you should see the service like this. At this point, a Service Bus WCF listener should also be registered in your namespace (you can check it in Azure Portal)
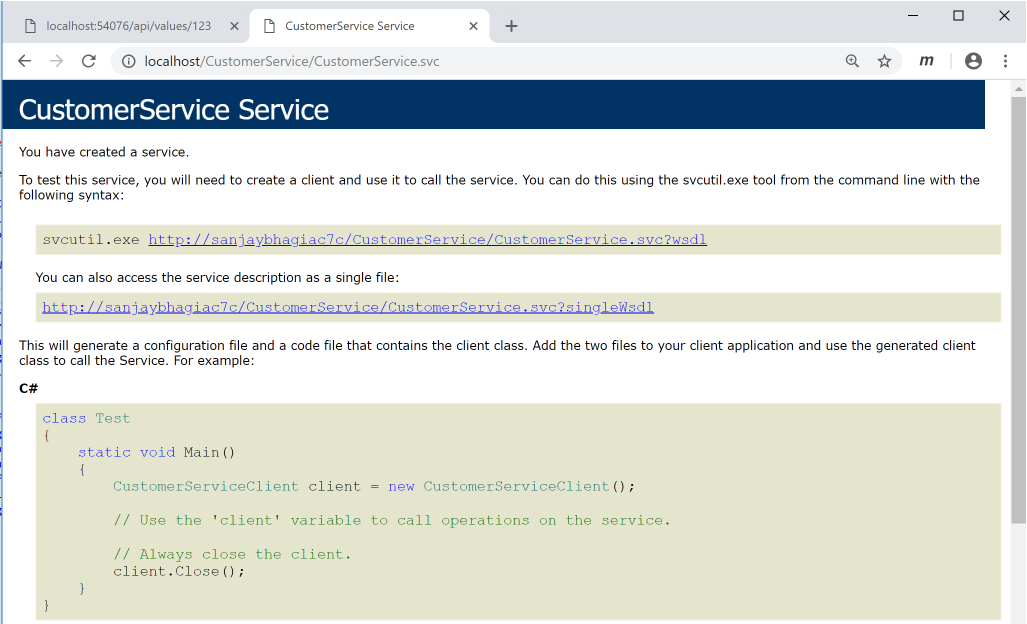
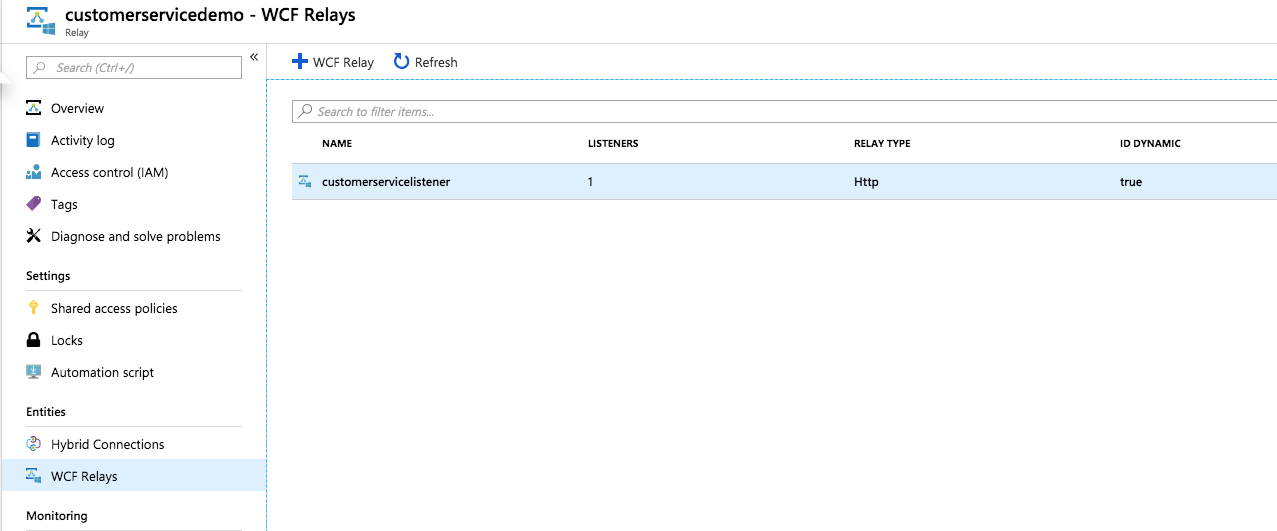
Now, let's start with the web application. I have created a vanilla ASP.NET Core Web API project (2.1). You can grab the source code from here (and clone it if you want to try it out yourself). Make sure you update settings in
After creating the project, I added following NuGet package
Nuget Packages:
- Microsoft.Azure.ServiceBus
In ValuesController, I have created a GetCustomerData method that takes in customerId as a string. In here, I'll make a call to WCF Service and return the response I receive from the service (the WCF service returns a dummy response)
[HttpGet("{customerId}")]
public async Task<string> GetCustomerData(string customerId)
Then moving forwards, I generate the token for Azur Service Bus Relay:
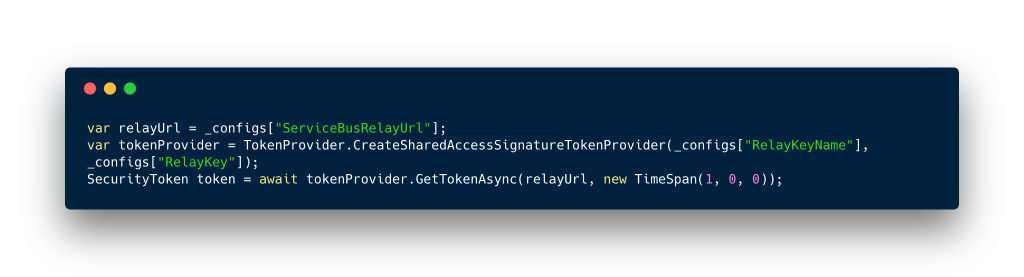
With this information, I construct the HTTP Post request like this:
using (var httpClient = new HttpClient()) | |
{ | |
//This header is required for Service Bus Relay | |
httpClient.DefaultRequestHeaders.Add("ServiceBusAuthorization", token.TokenValue); | |
//Here we setup the SOAP Action - schema: http://tempuri.org/<Interface>/<OperationName> | |
httpClient.DefaultRequestHeaders.Add("SOAPAction", "http://tempuri.org/ICustomerService/GetCustomerData"); | |
httpClient.DefaultRequestHeaders.Accept.Add(new System.Net.Http.Headers.MediaTypeWithQualityHeaderValue("text/xml")); | |
//Setup soap message body | |
string soapMessageBody = @"<soapenv:Envelope xmlns:soapenv=""http://schemas.xmlsoap.org/soap/envelope/"" xmlns:tem=""http://tempuri.org/""><soapenv:Header /><soapenv:Body><tem:GetCustomerData><tem:customerId>" + customerId + "</tem:customerId></tem:GetCustomerData></soapenv:Body></soapenv:Envelope>"; | |
var request = new HttpRequestMessage() | |
{ | |
RequestUri = new Uri(relayUrl), | |
Method = HttpMethod.Post, | |
Content = new StringContent(soapMessageBody, Encoding.UTF8) | |
}; | |
var response = await httpClient.SendAsync(request); | |
responseText = await response.Content.ReadAsStringAsync(); | |
} |
We are basically making
- ServiceBusAuthorization - Relay Token retrieved earlier
- SOAPAction - The Operation you want to call from WCF Service
- Accept header - text/
xml
Lastly, we need to set the body for the request - since this is a SOAP call, we need a
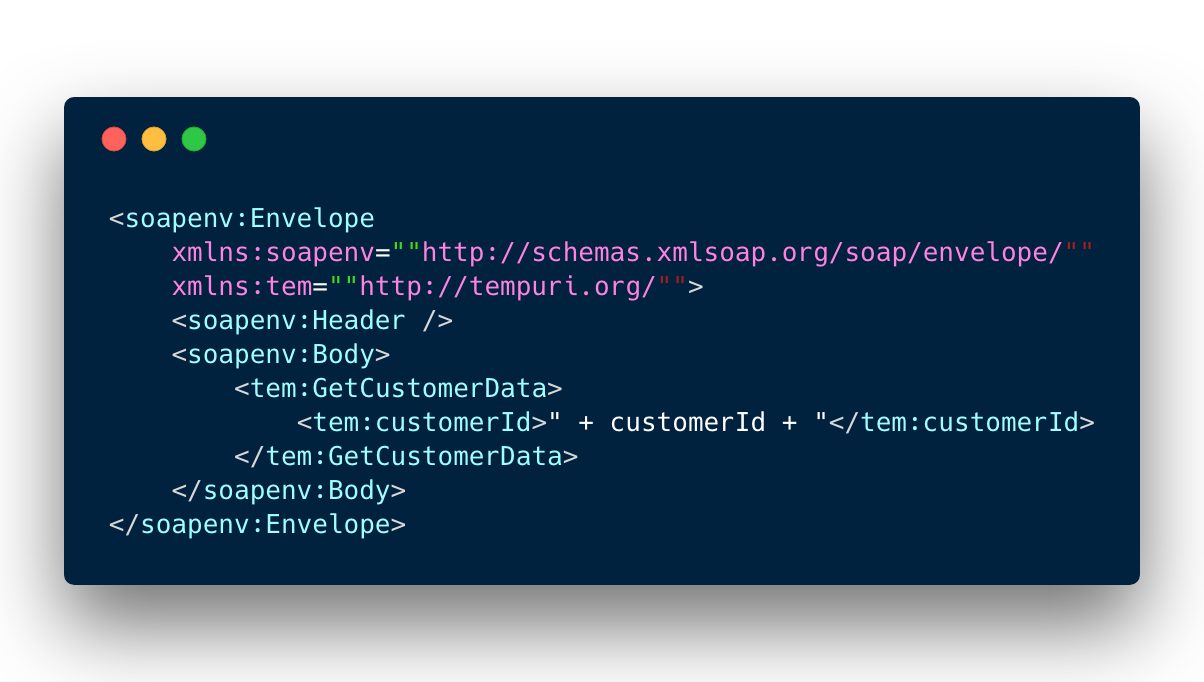
Press F5 and run the web application, pass in
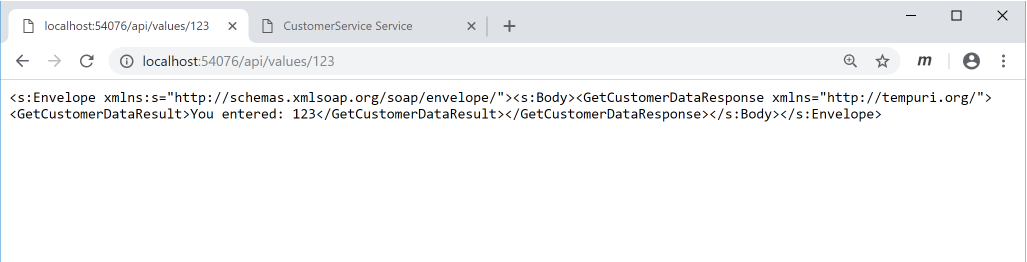
Have you tried to consume WCF Service from .NET Core application? How did it go for you? I would love to hear from you and would appreciate if you could suggest a better way of doing this as of today? I guess I don't need to tell you that I don't like using SOAP :)
Cheers.